What exactly is a [Software] Framework? In our quest to take down popular tech buzzwords, we’re going to tackle Software Frameworks.
Definition
A program that allows you to hook into itself to extend it.
That’s the one liner, but lets take it apart through an example that is your operating system.
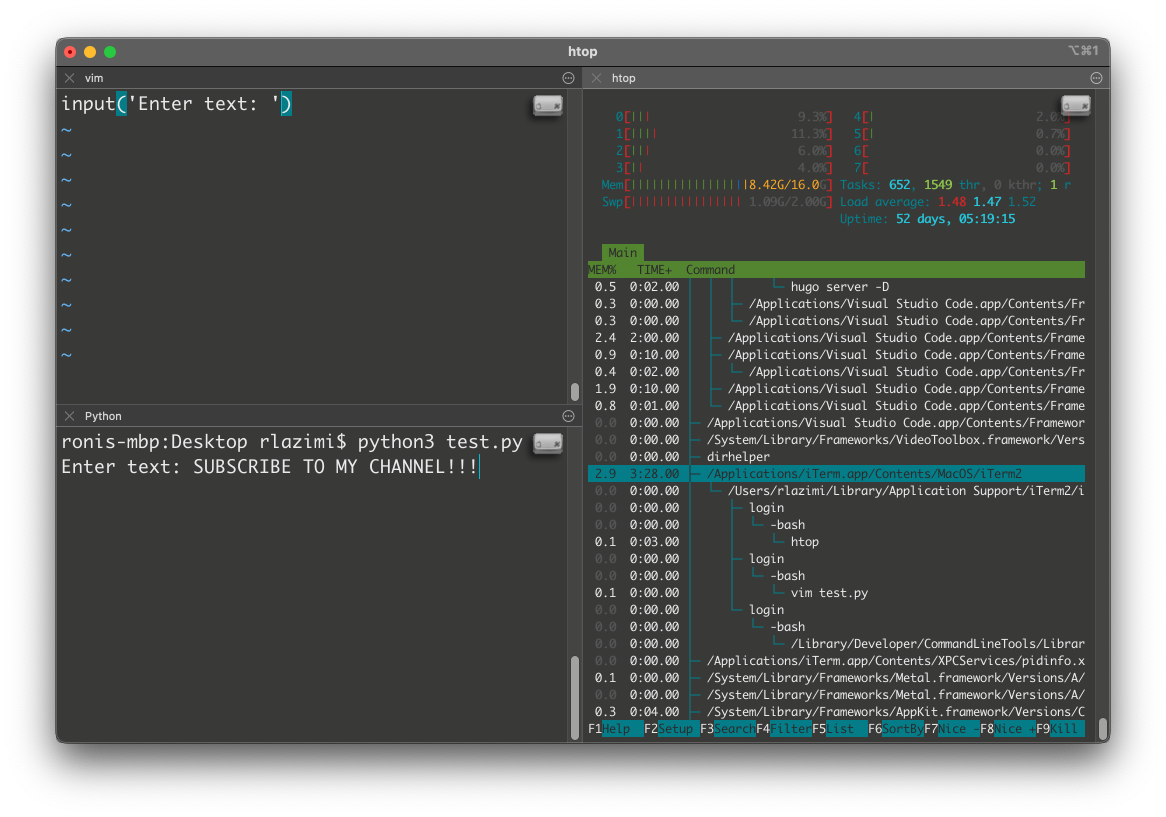
A running operating system is a process that provides a framework for running other processes. Each process can spawn child processes, which leads to a tree/heirarchy of processes that we can view through a command like htop
.
In the example, I’ve started two processes on the left (vim
and python3
), and on the right I’m using htop to view where those processes fall into the process tree in my machine.
Notice that vim and python are descendants of the iTerm2
process. iTerm2 is my hook into the existing process tree thats always running in my MacBook.
The Hook
The ability to hook into an underlying process [, engine, etc.] is a telltale sign that you’re dealing with a framework. In the operating system example mentioned earlier, the hook into the operating system framework was the terminal session.
Framework is a very broad word, so although every framework provides the idea of a hook, any specific hook will be structured according to the unique expectations set by the framework.
In the example of operating systems earlier, an OS can run a variety of processes, so the expectations it sets are not really clear. Let’s look at a more clear example of a framework with rigid expectations – Next.js.
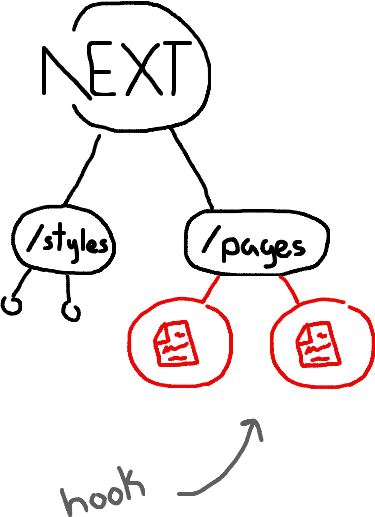
Next.js is a framework for creating websites, and is designed to be [beautifully] simple.
Next.js lets you create new pages in your site by simply creating files in the ./pages
directory at the root of your project, e.g.:
In other words, the Next.js HTML rendering engine allows you to hook into itself through the ./pages
directory, and on top of that it also expects you to export a React.js Component as a default export.
If you don’t know what that means: Node.js is another framework that many frameworks, like Next.js, are built on top of. Node enables JS files to be interpreted as modules, and modules can export specific functions that other files can import (you heard that right – modules are not native to JavaScript). A React Component is part of React.js’ framework for rendering HTML.
Below is the final product of my few lines of code.
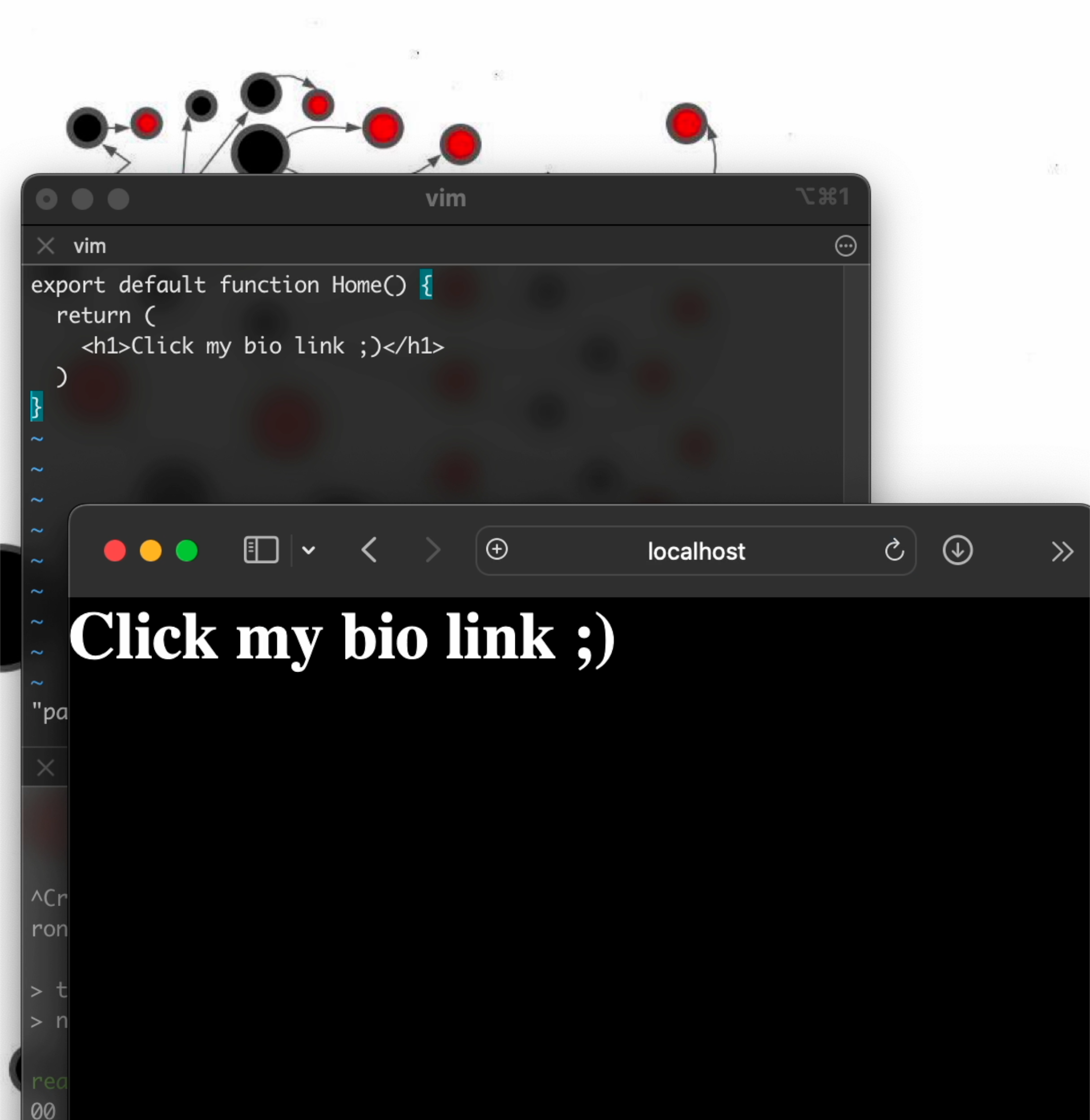
This is the power of frameworks.
And that’s about it for defining Frameworks, but I’d like to speak about one more concept that occurs as a consequence of using a Framework: Inversion of Control.
Inversion of Control
Inversion of Control refers to the fact that when you work with a framework, you’re moreso extending an existing program than you are architecting your own program.
The control of the running process is the framework’s, as opposed to yours.
Here’s an analogy. When you take your car to work: you are in control of your movement.
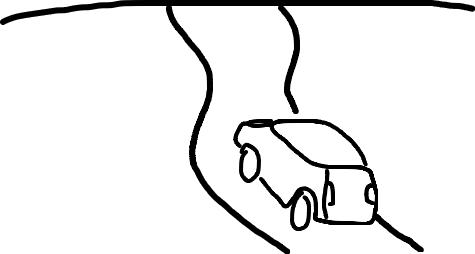
When you use the train: the train is in control of your movement. The public transport framework lets you hook into it by expecting you to be at a certain time and place (a train station at a specific time).
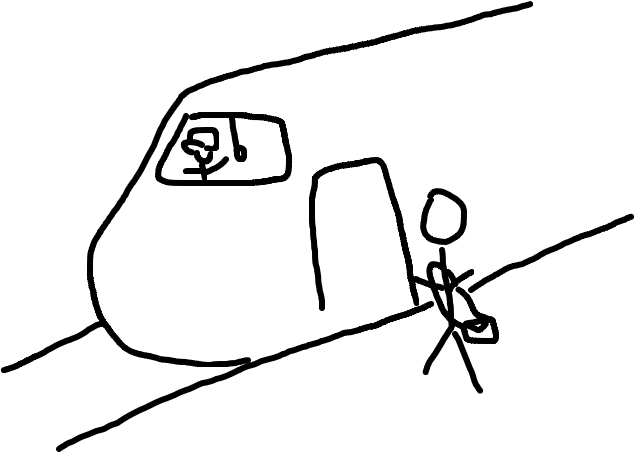
Library vs Framework
This is a bad/misinformed question - libraries and frameworks are independent concepts, so they’re not comparable.
The main difference, if you can call it that, is that a framework causes Inversion of Control.
Key Takeaways
- A framework exposes hooks. For example React.js is a program that manages a Component tree, and that lets you hook into that tree.
- Depending on the Framework, the hook needs to look as expected.
- A framework causes Inversion of Control. You are now writing code that extends an existing system as opposed to importing code like you would in a library.